GraphQL vs REST: Why Developers Are Making the Switch
The evolution of APIs from rigid endpoints to flexible queries.
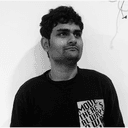
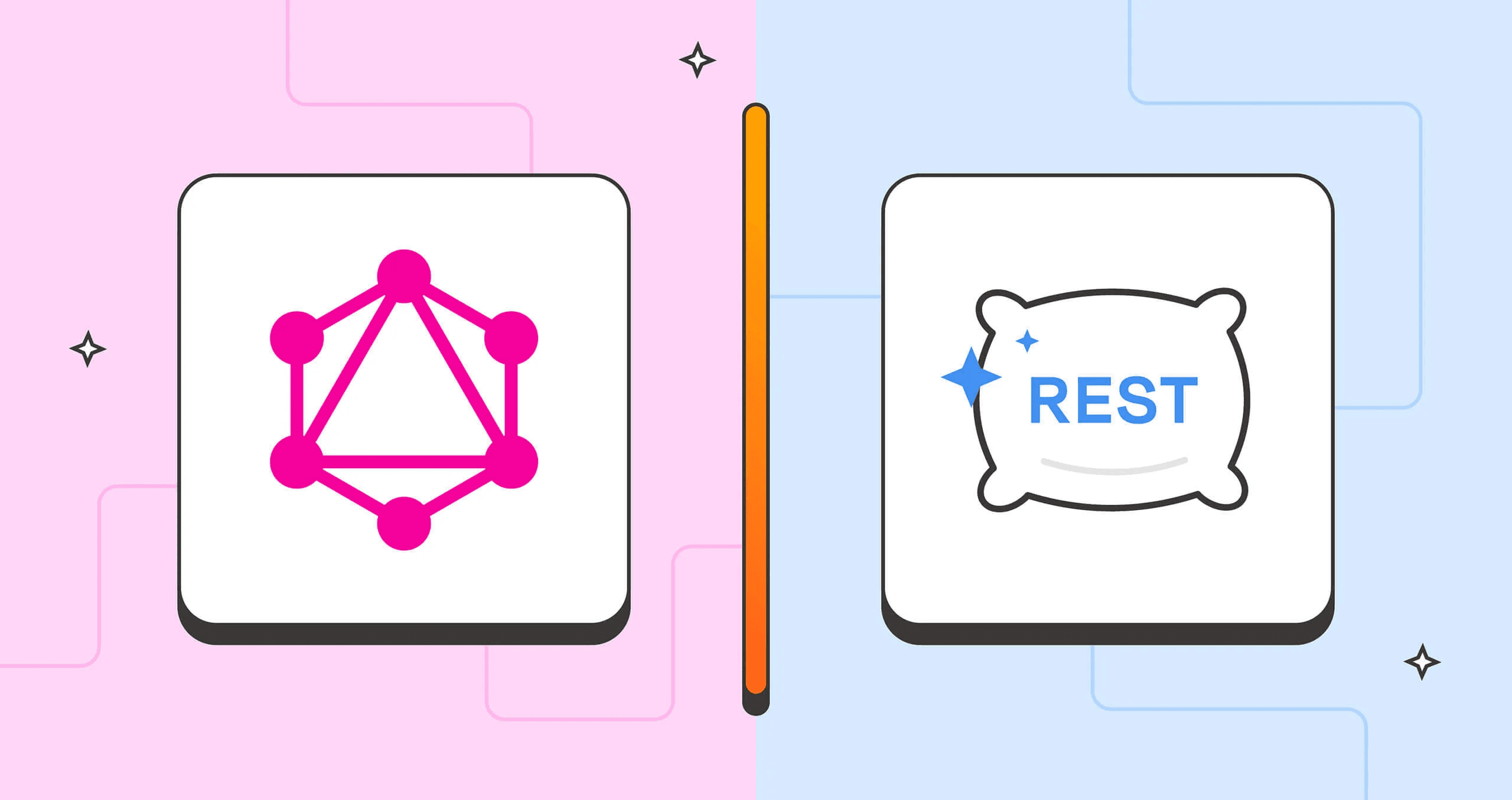
Introduction
In the ever-evolving landscape of web development, the architecture of APIs has undergone significant transformations. For more than a decade, REST (Representational State Transfer) dominated as the standard for building APIs. However, as applications grew more complex, developers began encountering limitations with REST. Enter GraphQL, developed by Facebook in 2012 and open-sourced in 2015, offering a more flexible query-based approach that addresses many REST pain points.
This article explores why many developers are switching from REST to GraphQL, examining their differences, performance implications, and real-world implementation stories.
Understanding REST
📦 The Building Blocks of REST
REST defines a set of constraints for building web services:
- Client-Server Architecture: Separation of concerns
- Statelessness: Each request contains all necessary information
- Cacheability: Responses define themselves as cacheable or non-cacheable
- Uniform Interface: Standardized resource identification and manipulation
In RESTful APIs, resources are identified by URLs, and operations use standard HTTP methods: GET, POST, PUT/PATCH, and DELETE.
A REST Example
Consider a blogging platform API:
GET /api/articles // Get all articles
GET /api/articles/123 // Get a specific article
POST /api/articles // Create a new article
PUT /api/articles/123 // Update an article
DELETE /api/articles/123 // Delete an article
GET /api/articles/123/comments // Get comments for an article
Limitations of REST
Despite its widespread adoption, REST faces several challenges:
- Overfetching: Returns fixed data structures, often providing more information than needed
- Underfetching: A single endpoint might not provide all data needed, requiring multiple requests
- Endpoint Proliferation: As applications evolve, the number of endpoints grows exponentially
- Versioning Challenges: Changes often require new API versions
- Limited Discoverability: Typically requires separate documentation
These limitations become more pronounced as applications grow in complexity and client needs diversify.
Enter GraphQL
🔍 A New Query Language for APIs
GraphQL provides a single endpoint that accepts queries specifying exactly what data clients need. The core principle is simple yet powerful: clients should request precisely the data they need, no more and no less.
The GraphQL Schema
At the heart of any GraphQL API is its schema, defining the capabilities using a type system:
type Article {
id: ID!
title: String!
content: String!
publishedDate: String!
author: User!
comments: [Comment!]!
tags: [String!]!
}
type User {
id: ID!
name: String!
email: String!
articles: [Article!]!
}
type Query {
article(id: ID!): Article
articles(limit: Int, offset: Int): [Article!]!
user(id: ID!): User
}
type Mutation {
createArticle(title: String!, content: String!): Article!
addComment(articleId: ID!, content: String!): Comment!
}
GraphQL Queries
Clients construct queries matching their data requirements:
query {
article(id: "123") {
title
content
author {
name
}
comments {
content
author {
name
}
}
}
}
The response mirrors the query structure, including only requested fields:
{
"data": {
"article": {
"title": "Understanding GraphQL",
"content": "GraphQL is a query language for APIs...",
"author": {
"name": "Jane Doe"
},
"comments": [
{
"content": "Great article!",
"author": {
"name": "John Smith"
}
}
]
}
}
}
Key Differences Between REST and GraphQL
📊 Comparative Analysis
Feature | REST | GraphQL |
---|---|---|
Endpoints | Multiple endpoints | Single endpoint |
Data Fetching | Fixed response structure | Client specifies fields |
Versioning | Typically requires explicit versioning | Evolve schema over time |
Caching | Natural HTTP caching | Requires custom solutions |
Error Handling | HTTP status codes | Errors in response body |
File Uploads | Straightforward with multipart/form-data | Requires additional specifications |
Discovery | External documentation | Built-in introspection |
Learning Curve | Lower | Higher |
The Request-Response Model
In REST, the server determines resource structure and endpoints. Clients must adapt and potentially make multiple requests.
GraphQL inverts this relationship, giving clients control to specify exactly what data they need in a single request. The server fulfills this request based on the schema's capabilities.
Type System and Introspection
GraphQL's type system provides a clear contract between client and server. Every field has a specific type, and the schema defines possible queries and response shapes.
Furthermore, GraphQL APIs are self-documenting through introspection. Clients can query the schema to discover available types, fields, and operations, enabling tools like GraphiQL for interactive API exploration.
Performance Considerations
⚡ Network Efficiency
GraphQL's ability to request exact data can significantly reduce network transfer. This optimization benefits mobile applications especially, where bandwidth may be limited or expensive.
This efficiency comes with a trade-off: flexible queries mean clients can potentially request very large datasets. Servers need to implement query complexity analysis and depth limiting to prevent resource-intensive queries.
Caching Strategies
REST leverages HTTP's caching mechanisms, with URLs serving as natural cache keys. This makes implementation straightforward at various levels: client, CDN, or proxies.
GraphQL presents more caching challenges due to its variable queries. Implementation strategies include:
- Client-Side Caching: Libraries like Apollo Client store objects by ID for reuse across queries
- Persisted Queries: Storing complex queries on the server, referenced by ID
- Field-Level Caching: Caching expensive field resolutions
- CDN Integration: Using automatic persisted queries (APQ) for caching common queries
These strategies often require additional infrastructure compared to REST's caching model.
When to Choose REST
🚦 Ideal Scenarios for REST
REST remains appropriate for many scenarios:
- Simple CRUD Applications: The resource-oriented approach provides clean, intuitive design
- Public APIs: REST's widespread adoption makes it accessible to more developers
- Caching-Critical Systems: Natural compatibility with HTTP caching
- File Operations: More straightforward handling of file uploads/downloads
- Microservices with Clear Boundaries: Clean API design with minimal cross-service data needs
REST Best Practices
Following these practices mitigates REST limitations:
- Consistent Resource Naming: Use plural nouns and consistent conventions
- Proper HTTP Method Usage: Leverage semantics correctly
- Comprehensive Documentation: Use OpenAPI/Swagger for interactive documentation
- Partial Responses: Support field selection through query parameters
- Compound Documents: Allow embedding related resources to reduce requests
When to Choose GraphQL
🌟 Ideal Scenarios for GraphQL
GraphQL excels in scenarios where REST struggles:
- Complex, Interconnected Data: Retrieve deeply nested or highly related data in one request
- Variable Client Requirements: Different clients (web, mobile, third-party) can request exactly what they need
- Rapid Product Iteration: Add new fields without breaking existing clients
- Aggregation APIs: Combine data from multiple services with a unified interface
- Real-Time Features: Subscriptions provide standardized real-time functionality
- Developer Experience Priority: Strong typing, self-documentation, and rich tooling
GraphQL Best Practices
Maximize benefits while mitigating challenges:
- Schema Design: Design around business domains, not database tables
- Pagination: Implement consistent patterns using cursor-based or limit/offset approaches
- N+1 Problem: Use DataLoader for query batching and optimization
- Security: Apply depth limiting, complexity analysis, and field-level authorization
- Schema Evolution: Use deprecation annotations for gradual transitions
Real-World Case Studies
🏆 Success Stories
GitHub
GitHub's transition to GraphQL for API v4 addressed several issues with their REST API:
- Multiple Requests Problem: Previously required chaining multiple API calls for related data
- Overfetching: REST endpoints returned excessive data for common use cases
- Version Management: Difficulty evolving API while maintaining compatibility
GitHub Engineering reported that GraphQL enabled them to build more efficient clients while giving developers precisely the data they needed. For common operations like displaying repository information with contributor details, GraphQL reduced multiple REST calls to a single query, significantly improving performance.
Their public GraphQL API now powers GitHub's own web interface and mobile applications, demonstrating confidence in the technology at scale.
Shopify
Shopify maintains both REST and GraphQL APIs, recognizing each has its place. Their GraphQL API particularly excels for complex storefront applications, where merchants display products, collections, customer information, and order details on a single page.
According to Shopify's engineering team:
"GraphQL gives our merchants' developers the flexibility to create unique experiences without requiring Shopify to build and maintain specialized endpoints for each use case."
This pragmatic approach shows how GraphQL can complement existing REST infrastructure rather than replacing it entirely.
Airbnb
Airbnb adopted GraphQL to solve server-side rendering challenges for complex React components. Their engineering team highlighted several benefits:
- Component-Driven Data Fetching: Components declare exact data needs
- Performance Improvements: Reduced network overhead through precise queries
- Developer Productivity: Simplified data access with stronger type guarantees
For Airbnb's listing pages—which combine property details, host information, reviews, and neighborhood data—GraphQL provided a 10x reduction in data transfer size compared to their previous REST implementation.
Netflix
Netflix adopted GraphQL to unify their API ecosystem across different device platforms:
- Platform Specialization: Different clients (TV, mobile, web) have vastly different data requirements
- API Consistency: GraphQL provided a unified interface across platforms
- Performance: Reduced bandwidth usage critical for streaming services
The company reported that GraphQL helped them deliver faster app startup times by eliminating unnecessary data transfers and reducing the number of round-trips between client and server.
Hybrid Approaches
Many organizations adopt hybrid approaches:
- GraphQL Gateway with REST Backends: A GraphQL server aggregates data from existing REST services
- REST for CRUD, GraphQL for Queries: Maintain REST for simple operations while using GraphQL for complex queries
- Progressive Migration: Gradually introduce GraphQL for new features or specific application sections
- Specialized APIs: Separate APIs for different purposes (REST for public APIs, GraphQL for internal use)
Implementation Challenges
🛠️ Common Obstacles and Solutions
Learning Curve
GraphQL introduces new concepts requiring adjustment for REST-familiar developers.
Solutions:
- Start with small, non-critical projects
- Leverage online courses and community resources
- Use code generation tools to reduce boilerplate
Performance Optimization
GraphQL's flexibility can strain backend resources without proper optimization.
Solutions:
- Implement DataLoader for batching and caching
- Use query complexity analysis to reject expensive queries
- Profile and optimize resolver performance
Authentication and Authorization
Implementing proper security at the field level requires careful consideration.
Solutions:
- Implement authentication at the transport layer
- Use directives or middleware for field-level authorization
- Apply depth limiting to prevent abuse
Migration Strategy
Transitioning existing REST APIs presents significant challenges.
Solutions:
- Implement GraphQL as a layer over existing services
- Migrate incrementally, starting with high-value endpoints
- Run both APIs in parallel during transition
The Future of APIs
🔮 Emerging Trends
Federation and Distributed GraphQL
GraphQL Federation allows teams to build and maintain separate GraphQL services combined into a unified graph. This approach aligns with microservice architectures, enabling team ownership of schema portions while providing a unified API.
Enhanced Type Systems
GraphQL's type system continues evolving with features like custom directives, input unions, and improved error handling, providing more expressive power for API designers.
Real-Time and Event-Driven APIs
The combination of GraphQL with WebSockets, Server-Sent Events, and event sourcing is creating new possibilities for real-time applications beyond basic subscriptions.
GraphQL for IoT and Edge Computing
GraphQL's efficient data transfer makes it well-suited for bandwidth-constrained environments like IoT networks or edge computing scenarios.
Conclusion
The choice between REST and GraphQL isn't binary—both have their place in modern API development. REST offers simplicity and broad compatibility, while GraphQL provides flexibility and efficiency for complex data requirements.
As we've seen from real-world implementations at companies like GitHub, Shopify, Airbnb, and Netflix, GraphQL can deliver tangible benefits:
- Reduced network requests and data transfer
- More flexible client development
- Improved developer experience
- Better documentation and discoverability
- Easier API evolution
However, these benefits come with implementation challenges that must be carefully addressed.
The trend is clear: clients demand more control over data consumption, and GraphQL meets this demand with its flexible, powerful approach. Whether building a new API or considering an upgrade to existing infrastructure, GraphQL deserves serious consideration as part of your API strategy.
Key Takeaways
- REST excels at simplicity, caching, and compatibility
- GraphQL shines with complex data, flexible queries, and reduced network requests
- Major companies have successfully adopted GraphQL to solve specific challenges
- Consider hybrid approaches that leverage both technologies' strengths
- Address implementation challenges with proper tooling and patterns